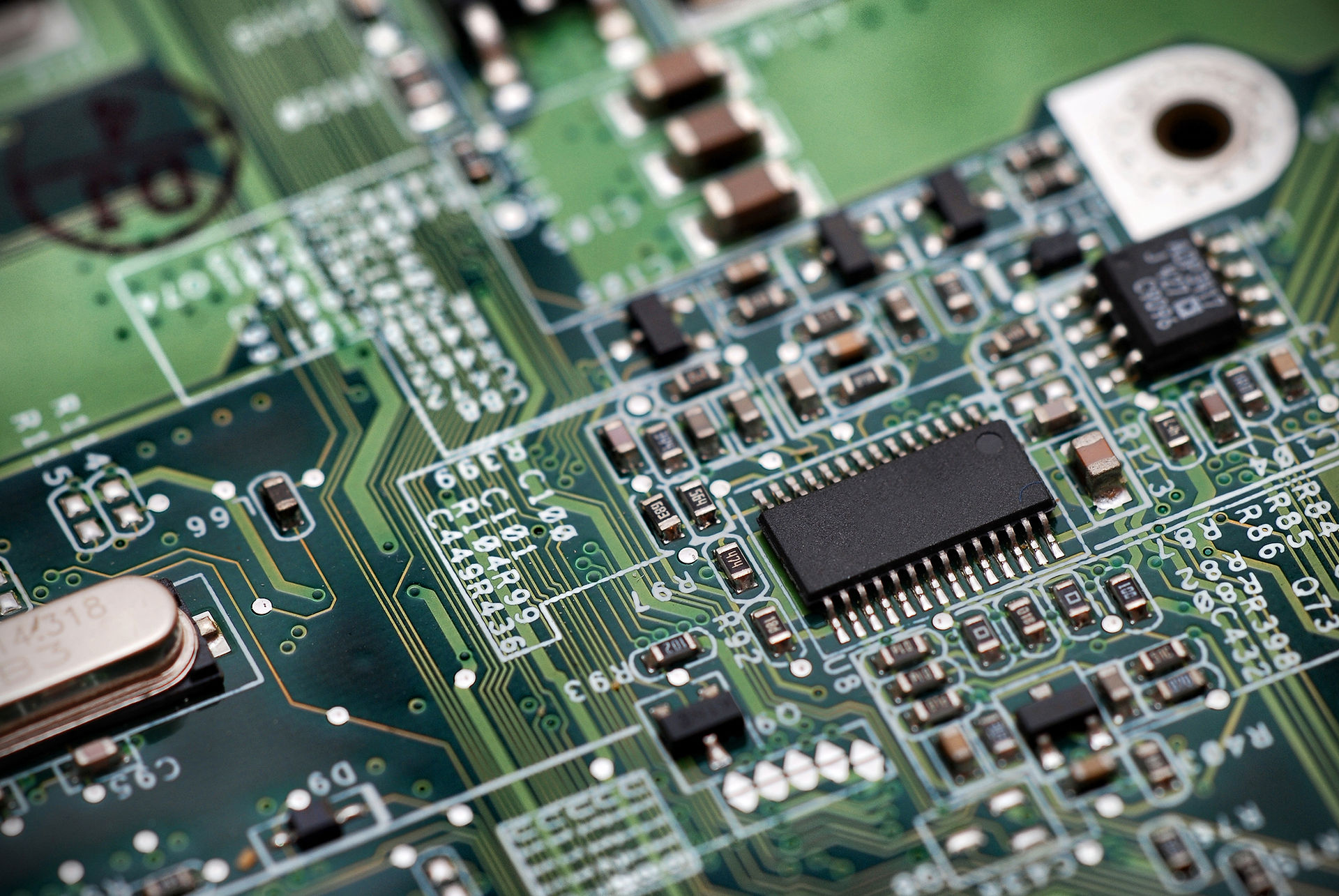
FIRST TECH CHALLANGE
Programming Tutorials
Java Programming Basics
Basic intro into the Java programming language. Contains how to format Java code, utilize variables and the different types of them. Also introduces the concepts of conditionals and loops.
Formatting
Comments are important for keeping organized code. Comments are parts of the code that won't run with the code and are instead used as notes on a program. You should be leaving comments in your code to describe how your code works! Comments are useful for showing your team members how your code works, and even for you to read later. It is important for your comments to be clear and descriptive so everyone who reads them can understand them.
Comments in Java:
//This is a comment!
/*
This is a multiline comment
it works over more than just a single line
*/
Indentation is important for keeping your code readable. After any line of code that requires an opening bracket, your code should be one tab over and after a closing bracket, you should remove that indent.
Your code should look like this:
if(condition)
{
statement;
}
In addition, there are many important decisions to make on how you will format your code. Your team will need to agree on how you want to format your code and you should remember to keep all of your team code consistent.
​
Here is a link to the official style guide for Java by Google
Variables and Types
Java has many different types of variables. Variables are used to store data that can be read from and written to.
Primitive data types are the most common type of variables and simply store a specified type of value. Here is a list of the most common primitive data types:
Int - Integers or ints store positive or negative non-decimal 32-bit integer numbers. You can perform math operations on them such as addition and subtraction.
int my_first_integer = 68;
Double - Similar to an integer doubles store 64-bit numeric values, however, unlike integers, doubles can store decimal values.
double my_first_double = 8.63673;
Float - Floats work similarly to doubles but only store 32-bit values.
float my_first_float = 38.387
Long - Longs store integer numbers like the int but they can store 64-bit integers instead of 32.
long my_first_long = 41582;
Short - Shorts store 16-bit integers.
short my_first_short = 7834758914732654;
Byte - Bytes store 8-bit integers.
byte my_first_byte = 562354;
Char - Chars store single characters.
//the charecter that you want to store should have single quotes around it
char the_first_charecter = 'w';
Boolean - Booleans store a single true or false (on or off) value.
boolean my_first_boolean = true;
Example Code For Variable:
//variable declaration
int x = 0;
//after a variable has been defined you can redefine it to change its value
x = 1;
//you can use the current value of a variable in the value you are setting
x = x + 1; //this can be shortened with x += 1 or x++;
//you can create variables of other data types in the same way
//you can also use variables in the definition of new variables
int y = x + 5;
These are some of the basics of variables but there is so much more you can do with them!
Note: another of the most common variable types you will use are Strings, but Strings are what are known as objects. We do a deep dive into the world of objects in our Object-Oriented Programming section.
Conditionals and Loops
Conditionals and loops allow for a more complex sequence of statements. They require a condition to be given as well as a series of statements started with a { and ended with a }
If statements will only run the code inside them if the given condition is true.
Example
//note that when testing a condition a double equal is used instead of a single
//a single equal sign is used to assign, a double equal sign is used to compare
if(x == 1)
{
x = 2;
}
In the above example if x is one, the code inside of the if statement will run, setting x equal to 2.
While loops will repeat the given code as long as the condition is met.
Example:
while(x == true) //you can also do while(x){} to see if x would return true or false
{
y++;
}
The above code will add 1 to y as long as x is true.
The do while loop works in a very similar way to the while loop, however, it will run the code one time before the condition is checked
Example:
do{
y++;
} while(!x);
//an exclamation point before a condition is known as the "not" operator
//it reverses whatever the condition is (true becomes false and false becomes true)
In this example, the code will at 1 to y and then keep doing so as long as x is false