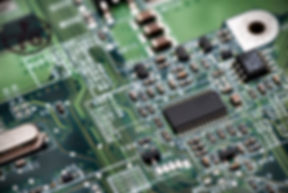
FIRST TECH CHALLANGE
Programming Tutorials
Object-Oriented Programming
Introduction to Object-Oriented Programming (OOP), the basis of most programming languages, and specifically Java
What is Object-Oriented Programming and how do we use it?
What is it?
Object-Oriented Programming (OOP) is a style of programming where you create multiple smaller occurrences of programs that act on their own. For example, if you were to make a Ball class with a size and color, you would be able to make a Ball object which you could modify separate from the overall Ball class. OOP is a major part of all programming and is the foundation of Java.
How do we use it?
We use OOP in a number of ways. One of these is how we have an object for our robot, which contains smaller objects for each part of that robot, such as an arm or the drive train. The most common type of Object is a string. All strings are different and can have different things done to them without changing the overall function of the String class. We can also use it to be a storage house for shared things. An example specific to FTC for our team is that we use multiple of the same functions for all autonomous programs. All of those functions are placed into the same class and object.
Classes
Classes are where you store your code, you can think of them as similar to a Google Doc but for your code. People can make copies of a Google Doc (objects) but they all would contain the same main information upon being copied (class information). Separating things into different classes can make your code a lot more organized and easy to read. For example, having a class for your intake mechanism, a class for your drive train, and classes for different autonomous/TeleOp programs really helps simplify things.
At the beginning of every FTC program we make, we start a file with this text to import the necessary methods and declare the class:
// This line just lets the app know where this code is located relative to other things
package org.firstinspires.ftc.teamcode;
// This line lets you use motors
import com.qualcomm.robotcore.hardware.DcMotor;
public class Robot
{
}
Whenever you make a class, the name of the file should match the class declared in the code. In this file, I declared the class to be named Robot, so I have to name the file Robot.java. Classes, while simple, are one of the most important parts of coding. An important note on classes is that they should have their first letter uppercase. While the code will still run if it isn't, this is the best practice.
Methods
Methods are a collection of statements to perform a task and return the result. For example, you could have a method which took two numbers and returned the result of the two of them multiplied together. FTC provides many methods for you to use but otherwise you make them yourself. For example, moving forward requires power being put into several motors so you could put all of those commands into a custom method. Then later in your code you would only have to write one line of code instead of many of the same. Here is an example of giving power to the drive motors:
public void setDriveMotorPowers(double power)
{
robot.leftDriveFront.setPower(power);
robot.rightDriveFront.setPower(power);
robot.leftDriveRear.setPower(power);
robot.rightDriveRear.setPower(power);
}
Fields
A field is a variable which is specific to a class/object and can have a setting to determine who can change that object's variable. Usually you will only be using the settings "public" or "private". "public" should be used if you want any code in your program to be able to change the field. "private" should be used if you only want the object to be able to change its variable. For example if you had a "Human" object with a "hairStyle" field, you would want to make it private so not just any random person could give you a haircut! Here is an example of how we use a field in our code:
private Hardware robot;
​
Notice the order of the field. It should follow the order of:
"Visibility setting" "Type" "Name of variable"
Inheritence
Inheritance is a concept unique to object-oriented programming. The idea is you can separate your program into several classes to simplify your code. For example, if I had a car class, it could have a DriveTrain class, and maybe a headlight class. For our robot, we separate the different mechanisms, autonomous programs, and features. Here is our list of classes that we used during Rover Ruckus (the 2018-2019 game), and most of them worked together.

When a class inherits another class, it receives all the members of the class. Constructor methods don't count as members, so they are not inherited. When a class is inherited, the class that inherits another class is often called a subclass or child class. Likewise, the class that is being inherited is called the super or parent class. Here are some diagrams of inheritance:

This diagram, from javatpoint.com/inheritance-in-java, shows the many ways inheritance can work. In type 1, it is very simple, with one parent class and one subclass. In type 2, ClassB inherits ClassA, while ClassC inherits ClassB. This can let you get into specifics of programs. Using our car example from earlier, we could have the overall car class, a DriveTrain class, and a wheel class. In type 3, ClassB inherits ClassA, and ClassC inherits ClassA, but they do not interact like in type 2. Using a car again, you have a car, and then a DriveTrain and AirConditioning class. They both inherit the Car class, but they don't need to inherit each other.
As to how to actually have a class be inherited, it is very simple, using the keyword extends. You add it in on the normal line where you name the program. The subclass always goes first. Here is an example with our Car:
public class AirConditioning extends Car {}